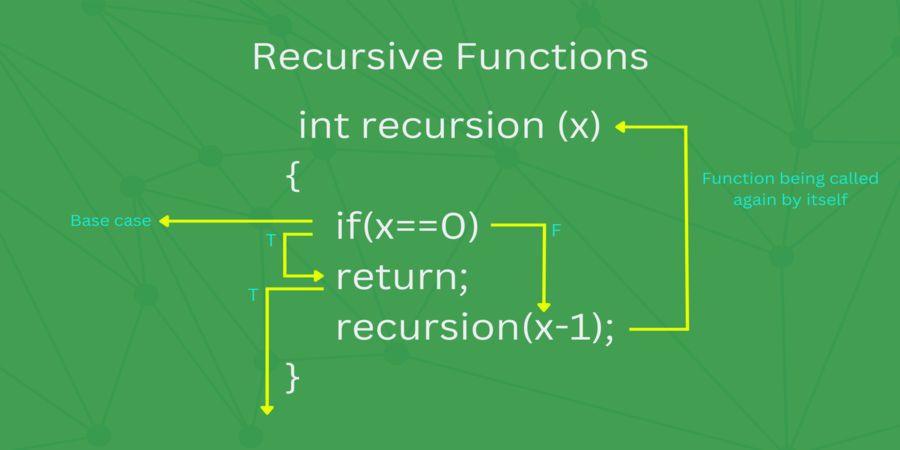
What is recursion and how is it used in programming?
Recursion is a concept in computer programming that allows a function or method to call itself. It is an important technique used in many programming languages, and it can be used to solve problems that are difficult or impossible to solve using traditional iterative approaches.
Recursion is a process where a function calls itself repeatedly until it reaches a base case, which is a condition that stops the recursion from continuing. In simpler terms, it is a way of solving a problem by breaking it down into smaller sub-problems, and then solving those sub-problems in a recursive manner until the solution is found.
Recursion can be used to solve a wide variety of problems, including searching, sorting, tree traversal, and mathematical calculations, among others. It is particularly useful for problems that involve complex data structures or algorithms, where a recursive approach can simplify the code and make it easier to understand.
Recursion is used in many programming languages, including C++, Java, Python, and JavaScript, among others. It is often used to simplify code and make it more readable, especially for complex problems. Some common examples of how recursion is used in programming are:
One of the most well-known examples of recursion is the calculation of the factorial of a number. In this case, the factorial of a number n is defined as the product of all integers from 1 to n. For example, the factorial of 5 is 5 x 4 x 3 x 2 x 1 = 120.
The recursive formula for calculating the factorial of a number is:
In this example, the function `factorial` calls itself with `n - 1` until it reaches the base case of `n == 0`. This approach is simpler and more elegant than an iterative solution.
Recursion is often used to traverse binary trees, which are data structures consisting of nodes that have two children each. There are three ways to traverse a binary tree: in-order, pre-order, and post-order.
In-order traversal visits the left subtree, then the root, then the right subtree. Pre-order traversal visits the root, then the left subtree, then the right subtree. Post-order traversal starts at the root and moves to the left child node before returning to the root.
Here is an example of a recursive implementation of in-order traversal:
In this example, the function `inorderTraversal` calls itself recursively to traverse the left and right subtrees until it reaches the base case of `root == nullptr`. This approach is more concise and readable than an iterative solution.
Recursion is also used in sorting algorithms such as merge sort. Divide and conquer with Merge Sort, which first sorts each half of an array independently before merging the results back together.
Here is an example of a recursive implementation of merge sort:
In this example, the function `mergeSort` calls itself recursively to sort the left and right halves of the array until it reaches the base case of `left >= right`. This approach is more efficient and easier to understand than an iterative solution.
One of the main benefits of recursion is that it can simplify code and make it more readable. Recursive solutions often involve fewer lines of code and can be more elegant than iterative solutions, especially for problems that involve complex data structures or algorithms.
Another benefit of recursion is that it can make code easier to debug and maintain. Recursive solutions are often easier to understand and modify than iterative solutions, because they break down problems into smaller sub-problems that can be independently tested and verified.
However, there are also some drawbacks to recursion that developers should be aware of. Recursive solutions can be less efficient than iterative solutions, especially for large datasets or complex problems. Recursive functions can also consume more memory and stack space than iterative functions, which can lead to performance issues or stack overflow errors.
Best Practices for Using Recursion
To use recursion effectively in programming, it is important to follow some best practices and guidelines. Some tips for using recursion include:
1. Always define a base case. What prevents the recursion from going further is the base case. Without a base case, a recursive function will continue to call itself indefinitely, which can lead to stack overflow errors or other issues.
2. Break down the problem into smaller sub-problems. Recursive solutions work by breaking down a problem into smaller sub-problems and solving them recursively. It is important to identify these subproblems and design a recursive algorithm that can solve them efficiently.
3. Use tail recursion when possible. Tail recursion is a technique where the recursive call is the last operation performed by the function. Tail recursion can be optimized by some compilers to use less stack space and improve performance.
4. Test and verify the algorithm. Recursive solutions can be complex and difficult to debug, so it is important to thoroughly test and verify the algorithm using a variety of test cases and edge cases.
Recursion is a powerful technique used in computer programming to solve problems that are difficult or impossible to solve using traditional iterative approaches. It allows a function or method to call itself repeatedly until it reaches a base case, which stops the recursion from continuing. Recursion is used in many programming languages and can be used to solve a wide variety of problems, including searching, sorting, tree traversal, and mathematical calculations. To use recursion effectively, it is important to follow best practices and guidelines, such as defining a base case, breaking down the problem into smaller sub-problems, and testing and verifying the algorithm.
Popular articles
Jun 08, 2023 07:51 AM
Jun 08, 2023 08:05 AM
Jun 08, 2023 03:04 AM
Jun 07, 2023 04:32 AM
Jun 05, 2023 06:41 AM
Comments (0)