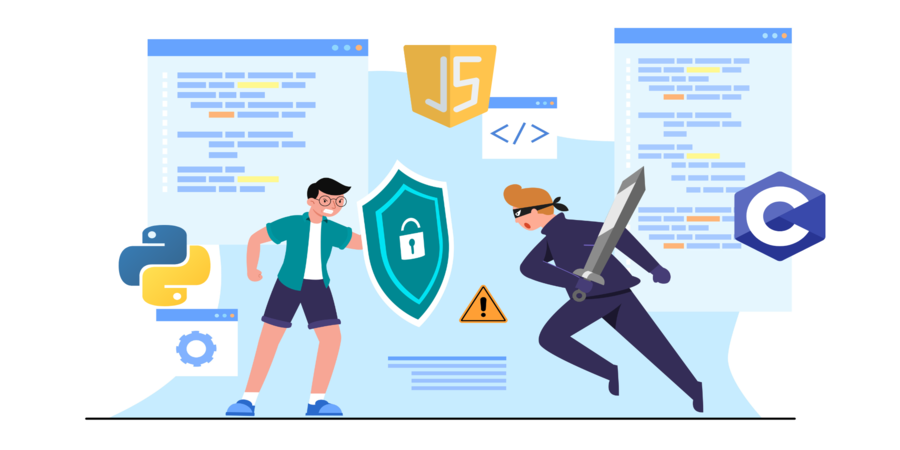
How do you implement security in Javascript python and C programming
In today's digital age, software security has become a crucial aspect of programming. Developers must consider security measures while writing code to protect users' data and prevent cyber attacks. In this article, we'll explore some best practices for implementing security in JavaScript, Python, and C programming and provide sample code for each language.
JavaScript is one of the most popular programming languages for web development, and security is a critical concern for web applications. Here are some tips for implementing security in JavaScript programming.
The eval() function in JavaScript can execute arbitrary code, which can be a significant security risk. When you use eval(), you're essentially allowing any user input to be executed as code, which can lead to things like SQL injection attacks or cross-site scripting (XSS) attacks. Instead of using eval(), you can use other functions like setTimeout() or setInterval() to execute code in a safer way.
For example, instead of using eval() to execute a string of code, you could use setTimeout():
When you're making requests to external APIs or sending data over the internet, it's important to use HTTPS instead of HTTP. HTTPS encrypts the data being transmitted, making it much more difficult for attackers to intercept and read. You can use the Fetch API in JavaScript to make requests over HTTPS:
One of the biggest security risks in web applications is user input. Users can submit malicious code or data that can cause all sorts of problems, from XSS attacks to database injection attacks. To prevent these attacks, it's important to validate all user input and sanitize any data that could be used maliciously.
For example, if you're accepting user input for a search query, you could use a regular expression to ensure that the input only contains letters and numbers:
Content Security Policy (CSP) is a security feature that helps prevent XSS attacks by allowing you to define which sources of content are allowed to be loaded on your web page. With CSP, you can specify which domains are allowed to load JavaScript, CSS, images, and other resources on your page. This can help prevent attackers from injecting malicious scripts into your page.
To use CSP in your JavaScript code, you can add a Content-Security-Policy header to your server response:
Finally, it's important to keep your JavaScript libraries up to date. Many libraries, such as jQuery or Angular, release security updates regularly that fix vulnerabilities and improve security. By keeping your libraries up to date, you can ensure that you're always using the most secure version of the library.
For example, if you're using jQuery, you can update to the latest version using the following code:
Python is a versatile programming language that is used in many applications, from web development to data analysis. Here are some tips for implementing security in Python programming.
When making requests to external APIs or sending data over the internet, it's important to use HTTPS instead of HTTP. HTTPS encrypts the data being transmitted, making it much more difficult for attackers to intercept and read.
To make requests over HTTPS in Python, you can use the requests library:
Just like in JavaScript, validating user input is crucial in Python programming. To prevent SQL injection or other malicious attacks, it's essential to sanitize and validate all user input.
For example, if you're accepting user input for a username, you could use the following code to ensure that the input only contains letters and numbers:
Python has many cryptography libraries that can be used to encrypt sensitive data or generate secure hashes. These libraries can be used to store user passwords securely or to encrypt sensitive data like credit card numbers.
For example, to generate a secure hash of a password, you can use the passlib library:
C programming is used in many critical applications, from operating systems to embedded systems. Here are some tips for implementing security in C programming.
Buffer overflows are a common vulnerability in C programming that can be exploited by attackers. To prevent buffer overflow attacks, it's important to use safe memory allocation functions like calloc() or malloc().
For example, to allocate memory for an array of integers, you could use the calloc() function:
C programming has many dangerous functions that can be used maliciously. It's important to avoid using functions like gets() or strcpy() that don't perform any bounds checking and can lead to buffer overflow attacks.
For example, instead of using gets() to read user input, you could use fgets() with a buffer size:
C programming has many encryption libraries that can be used to encrypt sensitive data or to generate secure hashes. These libraries can be used to store user passwords securely or to encrypt sensitive data like credit card numbers.
For example, to generate a secure hash of a password, you can use the OpenSSL library:
Implementing security measures in programming is a critical aspect of software development. By following the best practices outlined above, you can ensure that your code is secure and safe for users. Remember to use safe memory allocation functions, avoid dangerous functions, validate user input, use encryption libraries, and keep your libraries up to date. With these measures in place, you can protect your users' data and prevent cyber attacks.
Popular articles
Jun 08, 2023 07:51 AM
Jun 08, 2023 08:05 AM
Jun 08, 2023 03:04 AM
Jun 07, 2023 04:32 AM
Jun 05, 2023 06:41 AM
Comments (0)