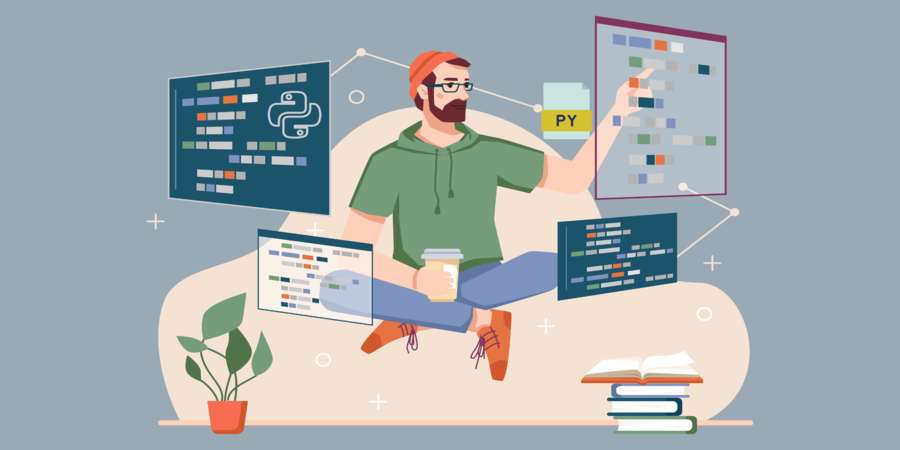
How do you manage memory in python programming
Python is a high-level, interpreted language that is widely used for web development, data analysis, machine learning, and more. One of the challenges of working with Python is managing memory usage effectively. Memory management is the process of allocating memory for objects, tracking which objects are in use, and freeing memory that is no longer needed. In this article, we will explore how memory management works in Python and techniques for managing memory in Python programming.
Python has an automatic memory management system called Garbage Collector, which is responsible for allocating and deallocating memory. Garbage Collector is a background process that runs periodically and tracks which objects are still in use. It frees the memory occupied by the objects that are no longer in use.
Python's Garbage Collector uses a reference counting mechanism to track which objects are in use. Reference counting is a technique that keeps track of how many references point to an object. If an object has zero references, it is no longer in use, and the Garbage Collector frees the memory occupied by the object.
Python also has a mechanism called cyclic garbage collector to handle objects that have circular references. Circular references occur when two or more objects reference each other, creating a loop. In this case, reference counting alone is not enough to determine which objects are in use. Cyclic garbage collector identifies circular references and frees the memory occupied by the objects that are no longer in use.
Certainly, here are some examples of how to apply the memory management techniques discussed earlier in Python programming:
In conclusion, managing memory is an essential part of Python programming. By following the techniques outlined in this article, you can optimize your code for better memory usage and performance. Avoid creating unnecessary objects, use generators instead of lists, use context managers, use slicing to create views of arrays, use del to free memory, and use a memory profiler to identify memory leaks. With these techniques, you can write Python code that is efficient
Popular articles
Jun 08, 2023 07:51 AM
Jun 08, 2023 08:05 AM
Jun 08, 2023 03:04 AM
Jun 07, 2023 04:32 AM
Jun 05, 2023 06:41 AM
Comments (0)