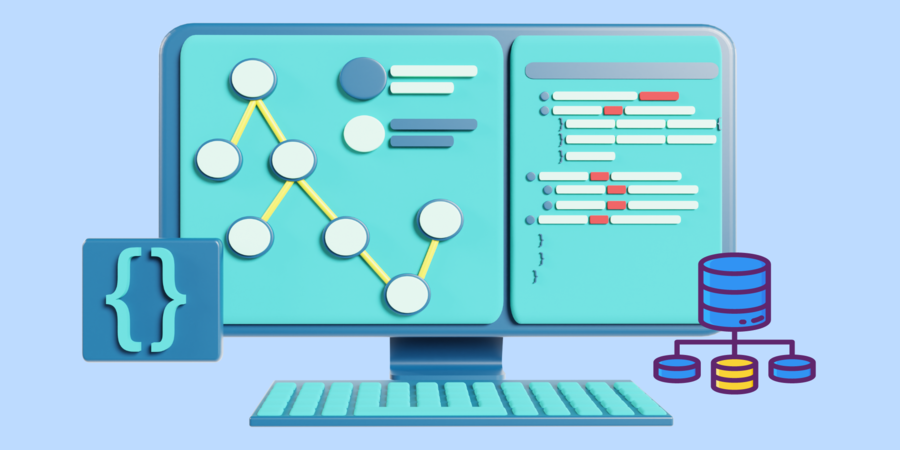
What is a data structure and how does it relate to programming
Data structure is a fundamental concept in computer science and programming. It refers to the way data is organized, stored, and accessed in a program. Data structures are essential for efficient algorithm design and optimization, making them a critical component of programming.
A data structure is a way of organizing data so that it can be used effectively. It is a collection of data values, the relationships between them, and the operations that can be performed on them. There are many different types of data structures, each with its own strengths and weaknesses.
For example, arrays are a common data structure used to store a collection of elements of the same data type. They provide efficient random access to elements and are ideal for situations where the size of the collection is fixed. However, they can be inefficient when it comes to inserting or deleting elements.
Linked lists are another data structure that can be used to store a collection of elements. Unlike arrays, linked lists can grow and shrink dynamically, making them more flexible. However, they are not as efficient when it comes to random access to elements.
Data structures are an essential part of programming, as they provide a way to store and manipulate data efficiently. Without data structures, programs would need to use basic variables and operations, making it difficult to write complex algorithms.
Data structures are used in programming in many ways, including:
One of the primary uses of data structures is to store data in a program. By organizing data into a structured format, it can be stored more efficiently and accessed more easily. This is particularly important when dealing with large amounts of data, such as in databases or complex algorithms.
Data structures are also essential for efficient algorithm design. By choosing the right data structure for a problem, it is possible to design algorithms that are more efficient and require less memory. For example, if a program needs to search for a particular element in a collection, using a hash table may be more efficient than using a linked list.
Data structures can also be used to optimize programs. By choosing the right data structure and algorithm, it is possible to reduce the time and memory required to execute a program. For example, using a binary search algorithm with a sorted array can significantly reduce the time required to search for an element.
Examples of Data Structures in Programming
Arrays are a collection of elements of the same data type that are stored in contiguous memory locations. They provide efficient random access to elements and are ideal for situations where the size of the collection is fixed.
Here is an example of an array in C programming:
Linked lists are a collection of elements that are linked together through pointers. Each element contains a data value and a pointer to the next element in the list. Linked lists can grow and shrink dynamically, making them more flexible than arrays.
Here is an example of a linked list in C programming:
Stacks are a data structure that operates on a "last-in, first-out" (LIFO) principle. Elements are added and removed from the "top" of the stack. Stacks are commonly used in programming for undo-redo features, where an action is pushed onto the stack when executed and popped off the stack when undone.
Here is an example of a stack implementation in Python:
Queues are a data structure that operates on a "first-in, first-out" (FIFO) principle. Elements are added to the "back" of the queue and removed from the "front". Queues are commonly used in programming for scheduling or buffering.
Here is an example of a queue implementation in Java:
In summary, data structures are a fundamental concept in programming that provide a way of organizing data so that it can be used effectively. Data structures are essential for efficient algorithm design and optimization, making them a critical component of programming. Understanding the different types of data structures and when to use them is an essential skill for any programmer. By choosing the right data structure for a problem, it is possible to design efficient and effective algorithms that can handle even the most complex of tasks.
Popular articles
Jun 08, 2023 07:51 AM
Jun 08, 2023 08:05 AM
Jun 08, 2023 03:04 AM
Jun 07, 2023 04:32 AM
Jun 05, 2023 06:41 AM
Comments (0)